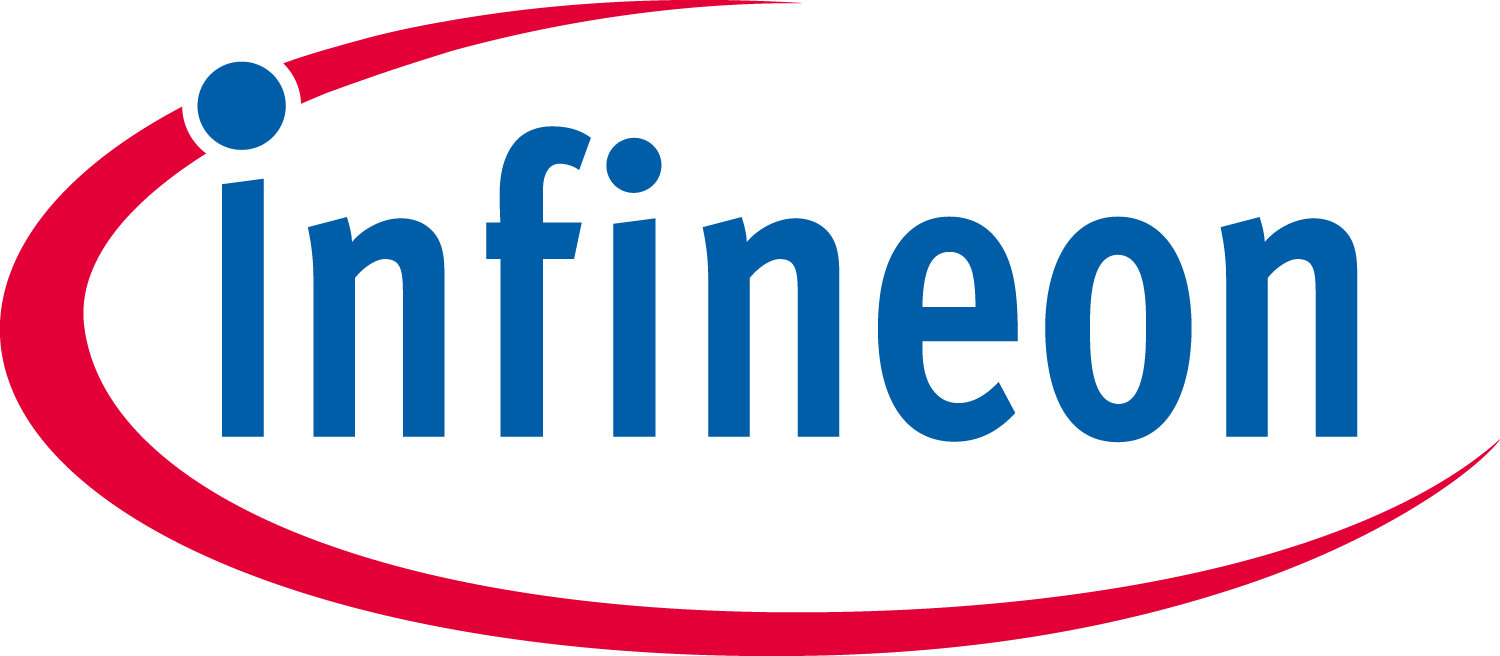 |
OPTIGA™ Trust M
Host Library Documentation
|
Go to the documentation of this file.
38 #ifndef _OPTIGA_CRYPT_H_
39 #define _OPTIGA_CRYPT_H_
58 #if defined (OPTIGA_CRYPT_RSA_ENCRYPT_ENABLED) || defined (OPTIGA_CRYPT_RSA_DECRYPT_ENABLED)
68 #if defined (OPTIGA_CRYPT_SYM_ENCRYPT_ENABLED) && defined (OPTIGA_CRYPT_SYM_DECRYPT_ENABLED)
72 #ifdef OPTIGA_CRYPT_SYM_GENERATE_KEY_ENABLED
91 #ifdef OPTIGA_COMMS_SHIELDED_CONNECTION
92 uint8_t protection_level;
95 uint8_t protocol_version;
96 #endif //OPTIGA_COMMS_SHIELDED_CONNECTION
103 #ifdef OPTIGA_COMMS_SHIELDED_CONNECTION
131 uint8_t parameter_type,
189 #ifdef OPTIGA_CRYPT_RANDOM_ENABLED
227 uint8_t * random_data,
228 uint16_t random_data_length);
229 #endif //OPTIGA_CRYPT_RANDOM_ENABLED
231 #ifdef OPTIGA_CRYPT_HASH_ENABLED
267 uint8_t source_of_data_to_hash,
268 const void * data_to_hash,
269 uint8_t * hash_output);
349 uint8_t source_of_data_to_hash,
350 const void * data_to_hash);
390 uint8_t * hash_output);
392 #endif //OPTIGA_CRYPT_HASH_ENABLED
395 #ifdef OPTIGA_CRYPT_ECC_GENERATE_KEYPAIR_ENABLED
442 bool_t export_private_key,
444 uint8_t * public_key,
445 uint16_t * public_key_length);
446 #endif //OPTIGA_CRYPT_ECC_GENERATE_KEYPAIR_ENABLED
448 #ifdef OPTIGA_CRYPT_ECDSA_SIGN_ENABLED
487 const uint8_t * digest,
488 uint8_t digest_length,
491 uint16_t * signature_length);
492 #endif //OPTIGA_CRYPT_ECDSA_SIGN_ENABLED
494 #ifdef OPTIGA_CRYPT_ECDSA_VERIFY_ENABLED
535 const uint8_t * digest,
536 uint8_t digest_length,
537 const uint8_t * signature,
538 uint16_t signature_length,
539 uint8_t public_key_source_type,
540 const void * public_key);
541 #endif //OPTIGA_CRYPT_ECDSA_VERIFY_ENABLED
543 #ifdef OPTIGA_CRYPT_ECDH_ENABLED
591 uint8_t * shared_secret);
592 #endif //OPTIGA_CRYPT_ECDH_ENABLED
594 #if defined (OPTIGA_CRYPT_TLS_PRF_SHA256_ENABLED) || defined (OPTIGA_CRYPT_TLS_PRF_SHA384_ENABLED) || defined (OPTIGA_CRYPT_TLS_PRF_SHA512_ENABLED)
648 const uint8_t * label,
649 uint16_t label_length,
650 const uint8_t * seed,
651 uint16_t seed_length,
652 uint16_t derived_key_length,
654 uint8_t * derived_key);
655 #endif // OPTIGA_CRYPT_TLS_PRF_SHA256_ENABLED || OPTIGA_CRYPT_TLS_PRF_SHA384_ENABLED || OPTIGA_CRYPT_TLS_PRF_SHA512_ENABLED
657 #ifdef OPTIGA_CRYPT_TLS_PRF_SHA256_ENABLED
708 const uint8_t * label,
709 uint16_t label_length,
710 const uint8_t * seed,
711 uint16_t seed_length,
712 uint16_t derived_key_length,
714 uint8_t * derived_key)
727 #endif // OPTIGA_CRYPT_TLS_PRF_SHA256_ENABLED
728 #ifdef OPTIGA_CRYPT_TLS_PRF_SHA384_ENABLED
778 const uint8_t * label,
779 uint16_t label_length,
780 const uint8_t * seed,
781 uint16_t seed_length,
782 uint16_t derived_key_length,
784 uint8_t * derived_key)
797 #endif // OPTIGA_CRYPT_TLS_PRF_SHA384_ENABLED
798 #ifdef OPTIGA_CRYPT_TLS_PRF_SHA512_ENABLED
848 const uint8_t * label,
849 uint16_t label_length,
850 const uint8_t * seed,
851 uint16_t seed_length,
852 uint16_t derived_key_length,
854 uint8_t * derived_key)
867 #endif //OPTIGA_CRYPT_TLS_PRF_SHA512_ENABLED
870 #ifdef OPTIGA_CRYPT_RSA_GENERATE_KEYPAIR_ENABLED
919 bool_t export_private_key,
921 uint8_t * public_key,
922 uint16_t * public_key_length);
923 #endif //OPTIGA_CRYPT_RSA_GENERATE_KEYPAIR_ENABLED
926 #ifdef OPTIGA_CRYPT_RSA_SIGN_ENABLED
966 const uint8_t * digest,
967 uint8_t digest_length,
970 uint16_t * signature_length,
971 uint16_t salt_length);
972 #endif //OPTIGA_CRYPT_RSA_SIGN_ENABLED
974 #ifdef OPTIGA_CRYPT_RSA_VERIFY_ENABLED
1018 const uint8_t * digest,
1019 uint8_t digest_length,
1020 const uint8_t * signature,
1021 uint16_t signature_length,
1022 uint8_t public_key_source_type,
1023 const void * public_key,
1024 uint16_t salt_length);
1025 #endif //OPTIGA_CRYPT_RSA_VERIFY_ENABLED
1027 #ifdef OPTIGA_CRYPT_RSA_PRE_MASTER_SECRET_ENABLED
1072 const uint8_t * optional_data,
1073 uint16_t optional_data_length,
1074 uint16_t pre_master_secret_length);
1075 #endif //OPTIGA_CRYPT_RSA_PRE_MASTER_SECRET_ENABLED
1077 #ifdef OPTIGA_CRYPT_RSA_ENCRYPT_ENABLED
1126 const uint8_t * message,
1127 uint16_t message_length,
1128 const uint8_t * label,
1129 uint16_t label_length,
1130 uint8_t public_key_source_type,
1131 const void * public_key,
1132 uint8_t * encrypted_message,
1133 uint16_t * encrypted_message_length);
1181 const uint8_t * label,
1182 uint16_t label_length,
1183 uint8_t public_key_source_type,
1184 const void * public_key,
1185 uint8_t * encrypted_message,
1186 uint16_t * encrypted_message_length);
1188 #endif //OPTIGA_CRYPT_RSA_ENCRYPT_ENABLED
1190 #ifdef OPTIGA_CRYPT_RSA_DECRYPT_ENABLED
1234 const uint8_t * encrypted_message,
1235 uint16_t encrypted_message_length,
1236 const uint8_t * label,
1237 uint16_t label_length,
1240 uint16_t * message_length);
1281 const uint8_t * encrypted_message,
1282 uint16_t encrypted_message_length,
1283 const uint8_t * label,
1284 uint16_t label_length,
1287 #endif //OPTIGA_CRYPT_RSA_DECRYPT_ENABLED
1289 #ifdef OPTIGA_CRYPT_SYM_ENCRYPT_ENABLED
1346 const uint8_t * plain_data,
1347 uint32_t plain_data_length,
1350 const uint8_t * associated_data,
1351 uint16_t associated_data_length,
1352 uint8_t * encrypted_data,
1353 uint32_t * encrypted_data_length);
1398 const uint8_t * plain_data,
1399 uint32_t plain_data_length,
1400 uint8_t * encrypted_data,
1401 uint32_t * encrypted_data_length);
1462 const uint8_t * plain_data,
1463 uint32_t plain_data_length,
1466 const uint8_t * associated_data,
1467 uint16_t associated_data_length,
1468 uint16_t total_plain_data_length,
1469 uint8_t * encrypted_data,
1470 uint32_t * encrypted_data_length);
1514 const uint8_t * plain_data,
1515 uint32_t plain_data_length,
1516 uint8_t * encrypted_data,
1517 uint32_t * encrypted_data_length);
1562 const uint8_t * plain_data,
1563 uint32_t plain_data_length,
1564 uint8_t * encrypted_data,
1565 uint32_t * encrypted_data_length);
1566 #endif //OPTIGA_CRYPT_SYM_ENCRYPT_ENABLED
1568 #ifdef OPTIGA_CRYPT_SYM_DECRYPT_ENABLED
1625 const uint8_t * encrypted_data,
1626 uint32_t encrypted_data_length,
1629 const uint8_t * associated_data,
1630 uint16_t associated_data_length,
1631 uint8_t * plain_data,
1632 uint32_t * plain_data_length);
1676 const uint8_t * encrypted_data,
1677 uint32_t encrypted_data_length,
1678 uint8_t * plain_data,
1679 uint32_t * plain_data_length);
1741 const uint8_t * encrypted_data,
1742 uint32_t encrypted_data_length,
1745 const uint8_t * associated_data,
1746 uint16_t associated_data_length,
1747 uint16_t total_encrypted_data_length,
1748 uint8_t * plain_data,
1749 uint32_t * plain_data_length);
1793 const uint8_t * encrypted_data,
1794 uint32_t encrypted_data_length,
1795 uint8_t * plain_data,
1796 uint32_t * plain_data_length);
1841 const uint8_t * encrypted_data,
1842 uint32_t encrypted_data_length,
1843 uint8_t * plain_data,
1844 uint32_t * plain_data_length);
1845 #endif //OPTIGA_CRYPT_SYM_DECRYPT_ENABLED
1848 #ifdef OPTIGA_CRYPT_HMAC_ENABLED
1895 const uint8_t * input_data,
1896 uint32_t input_data_length,
1898 uint32_t * mac_length);
1944 const uint8_t * input_data,
1945 uint32_t input_data_length);
1984 const uint8_t * input_data,
1985 uint32_t input_data_length);
2030 const uint8_t * input_data,
2031 uint32_t input_data_length,
2033 uint32_t * mac_length);
2034 #endif //OPTIGA_CRYPT_HMAC_ENABLED
2036 #ifdef OPTIGA_CRYPT_HKDF_ENABLED
2089 const uint8_t * salt,
2090 uint16_t salt_length,
2091 const uint8_t * info,
2092 uint16_t info_length,
2093 uint16_t derived_key_length,
2095 uint8_t * derived_key);
2147 const uint8_t * salt,
2148 uint16_t salt_length,
2149 const uint8_t * info,
2150 uint16_t info_length,
2151 uint16_t derived_key_length,
2153 uint8_t * derived_key)
2218 const uint8_t * salt,
2219 uint16_t salt_length,
2220 const uint8_t * info,
2221 uint16_t info_length,
2222 uint16_t derived_key_length,
2224 uint8_t * derived_key)
2289 const uint8_t * salt,
2290 uint16_t salt_length,
2291 const uint8_t * info,
2292 uint16_t info_length,
2293 uint16_t derived_key_length,
2295 uint8_t * derived_key)
2308 #endif //OPTIGA_CRYPT_HKDF_ENABLED
2310 #ifdef OPTIGA_CRYPT_SYM_GENERATE_KEY_ENABLED
2353 bool_t export_symmetric_key,
2354 void * symmetric_key);
2355 #endif //OPTIGA_CRYPT_SYM_GENERATE_KEY_ENABLED
2357 #ifdef OPTIGA_CRYPT_GENERATE_AUTH_CODE_ENABLED
2402 const uint8_t * optional_data,
2403 uint16_t optional_data_length,
2404 uint8_t * random_data,
2405 uint16_t random_data_length);
2406 #endif //OPTIGA_CRYPT_GENERATE_AUTH_CODE_ENABLED
2408 #ifdef OPTIGA_CRYPT_HMAC_VERIFY_ENABLED
2456 const uint8_t * input_data,
2457 uint32_t input_data_length,
2458 const uint8_t * hmac,
2459 uint32_t hmac_length);
2460 #endif // OPTIGA_CRYPT_HMAC_VERIFY_ENABLED
2461 #ifdef OPTIGA_CRYPT_CLEAR_AUTO_STATE_ENABLED
2492 #endif //OPTIGA_CRYPT_CLEAR_AUTO_STATE_ENABLED
2519 #ifdef OPTIGA_COMMS_SHIELDED_CONNECTION
2520 #define OPTIGA_CRYPT_SET_COMMS_PROTECTION_LEVEL(p_instance, protection_level) \
2522 optiga_crypt_set_comms_params(p_instance, \
2523 OPTIGA_COMMS_PROTECTION_LEVEL, \
2527 #define OPTIGA_CRYPT_SET_COMMS_PROTECTION_LEVEL(p_instance, protection_level) {}
2547 #ifdef OPTIGA_COMMS_SHIELDED_CONNECTION
2548 #define OPTIGA_CRYPT_SET_COMMS_PROTOCOL_VERSION(p_instance, version) \
2550 optiga_crypt_set_comms_params(p_instance, \
2551 OPTIGA_COMMS_PROTOCOL_VERSION, \
2555 #define OPTIGA_CRYPT_SET_COMMS_PROTOCOL_VERSION(p_instance, version) {}
Specifies the data structure for ecdh secret generation.
Specifies the data structure for symmetric encrypt and decrypt.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hkdf(optiga_crypt_t *me, optiga_hkdf_type_t type, uint16_t secret, const uint8_t *salt, uint16_t salt_length, const uint8_t *info, uint16_t info_length, uint16_t derived_key_length, bool_t export_to_host, uint8_t *derived_key)
Derives a key or shared secret using HKDF operation from the secret stored in OPTIGA.
LIBRARY_EXPORTS optiga_crypt_t * optiga_crypt_create(uint8_t optiga_instance_id, callback_handler_t handler, void *caller_context)
Create an instance of optiga_crypt_t.
_STATIC_INLINE LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_tls_prf_sha256(optiga_crypt_t *me, uint16_t secret, const uint8_t *label, uint16_t label_length, const uint8_t *seed, uint16_t seed_length, uint16_t derived_key_length, bool_t export_to_host, uint8_t *derived_key)
Derives a key using TLS PRF SHA256.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_symmetric_generate_key(optiga_crypt_t *me, optiga_symmetric_key_type_t key_type, uint8_t key_usage, bool_t export_symmetric_key, void *symmetric_key)
Generates a symmetric key using OPTIGA.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_generate_auth_code(optiga_crypt_t *me, optiga_rng_type_t rng_type, const uint8_t *optional_data, uint16_t optional_data_length, uint8_t *random_data, uint16_t random_data_length)
Generates a random number using OPTIGA and stores the same in acquired session context at OPTIGA.
union optiga_crypt_params optiga_crypt_params_t
union for OPTIGA crypt parameters
enum optiga_rsa_key_type optiga_rsa_key_type_t
Specifies the RSA key type in OPTIGA.
struct optiga_cmd optiga_cmd_t
OPTIGA command instance structure type.
optiga_encrypt_sym_params_t optiga_symmetric_enc_dec_params
derive key params
enum optiga_rsa_signature_scheme optiga_rsa_signature_scheme_t
Specifies the RSA signature schemes type in OPTIGA.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_rsa_encrypt_message(optiga_crypt_t *me, optiga_rsa_encryption_scheme_t encryption_scheme, const uint8_t *message, uint16_t message_length, const uint8_t *label, uint16_t label_length, uint8_t public_key_source_type, const void *public_key, uint8_t *encrypted_message, uint16_t *encrypted_message_length)
Encrypts message using RSA public key.
@ OPTIGA_HKDF_SHA_384
Key derivation using HKDF-SHA384.
enum optiga_hkdf_type optiga_hkdf_type_t
Specifies the HKDF key derivation types in OPTIGA.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hmac_finalize(optiga_crypt_t *me, const uint8_t *input_data, uint32_t input_data_length, uint8_t *mac, uint32_t *mac_length)
Generates HMAC on the input message using input secret from OPTIGA and exports the finalized HMAC to ...
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_random(optiga_crypt_t *me, optiga_rng_type_t rng_type, uint8_t *random_data, uint16_t random_data_length)
Generates a random number.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_rsa_decrypt_and_store(optiga_crypt_t *me, optiga_rsa_encryption_scheme_t encryption_scheme, const uint8_t *encrypted_message, uint16_t encrypted_message_length, const uint8_t *label, uint16_t label_length, optiga_key_id_t private_key)
Decrypts input data using OPTIGA private key and stores it in a OPTIGA session.
enum optiga_symmetric_encryption_mode optiga_symmetric_encryption_mode_t
Specifies the symmetric encryption schemes type in OPTIGA.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_ecdh(optiga_crypt_t *me, optiga_key_id_t private_key, public_key_from_host_t *public_key, bool_t export_to_host, uint8_t *shared_secret)
Calculates the shared secret using ECDH algorithm.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hmac_start(optiga_crypt_t *me, optiga_hmac_type_t type, uint16_t secret, const uint8_t *input_data, uint32_t input_data_length)
Initiates a HMAC generation sequence for the input data using input secret from OPTIGA.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hmac_update(optiga_crypt_t *me, const uint8_t *input_data, uint32_t input_data_length)
Generates HMAC on the input message using input secret from OPTIGA, update the previously generated H...
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_rsa_generate_pre_master_secret(optiga_crypt_t *me, const uint8_t *optional_data, uint16_t optional_data_length, uint16_t pre_master_secret_length)
Generates a pre-master secret.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_tls_prf(optiga_crypt_t *me, optiga_tls_prf_type_t type, uint16_t secret, const uint8_t *label, uint16_t label_length, const uint8_t *seed, uint16_t seed_length, uint16_t derived_key_length, bool_t export_to_host, uint8_t *derived_key)
Derives a key.
optiga_gen_keypair_params_t optiga_gen_keypair_params
get key pair params
Specifies the structure to the Hash context details managed by OPTIGA.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_symmetric_encrypt_ecb(optiga_crypt_t *me, optiga_key_id_t symmetric_key_oid, const uint8_t *plain_data, uint32_t plain_data_length, uint8_t *encrypted_data, uint32_t *encrypted_data_length)
Encrypt the data using symmetric encryption scheme using ECB mode of operation.
Specifies the data structure of random generation.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hmac(optiga_crypt_t *me, optiga_hmac_type_t type, uint16_t secret, const uint8_t *input_data, uint32_t input_data_length, uint8_t *mac, uint32_t *mac_length)
Generates HMAC on the input message using input secret from OPTIGA and exports the generated HMAC to ...
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_symmetric_decrypt_start(optiga_crypt_t *me, optiga_symmetric_encryption_mode_t encryption_mode, optiga_key_id_t symmetric_key_oid, const uint8_t *encrypted_data, uint32_t encrypted_data_length, const uint8_t *iv, uint16_t iv_length, const uint8_t *associated_data, uint16_t associated_data_length, uint16_t total_encrypted_data_length, uint8_t *plain_data, uint32_t *plain_data_length)
Initiate symmetric decryption sequence for input data using symmetric key from OPTIGA.
enum optiga_ecc_curve optiga_ecc_curve_t
Specifies the key curve type in OPTIGA.
enum optiga_hash_type optiga_hash_type_t
Specifies the hashing algorithm type in OPTIGA.
optiga_crypt_params_t params
Details/references (pointers) to the Application Inputs.
void * caller_context
Caller context.
Specifies the data structure for symmetric generate key.
Specifies the data structure of the Public Key details (key, size and type)
@ OPTIGA_TLS12_PRF_SHA_256
Key derivation using TLSv1.2 PRF SHA256.
enum optiga_tls_prf_type optiga_tls_prf_type_t
Specifies the key derivation types.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_rsa_decrypt_and_export(optiga_crypt_t *me, optiga_rsa_encryption_scheme_t encryption_scheme, const uint8_t *encrypted_message, uint16_t encrypted_message_length, const uint8_t *label, uint16_t label_length, optiga_key_id_t private_key, uint8_t *message, uint16_t *message_length)
Decrypts input data using OPTIGA private key and export it to the host.
optiga_calc_sign_params_t optiga_calc_sign_params
calc sign params
optiga_encrypt_asym_params_t optiga_encrypt_asym_params
asymmetric encryption params
enum optiga_rsa_encryption_scheme optiga_rsa_encryption_scheme_t
Specifies the RSA encryption schemes.
enum optiga_rng_type optiga_rng_type_t
Specifies the random generation types.
OPTIGA crypt instance structure.
Specifies the data structure of calculate hash.
optiga_verify_sign_params_t optiga_verify_sign_params
verify sign params
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_rsa_generate_keypair(optiga_crypt_t *me, optiga_rsa_key_type_t key_type, uint8_t key_usage, bool_t export_private_key, void *private_key, uint8_t *public_key, uint16_t *public_key_length)
Generates a key pair based on RSA key type.
@ OPTIGA_HKDF_SHA_256
Key derivation using HKDF-SHA256.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_symmetric_decrypt(optiga_crypt_t *me, optiga_symmetric_encryption_mode_t encryption_mode, optiga_key_id_t symmetric_key_oid, const uint8_t *encrypted_data, uint32_t encrypted_data_length, const uint8_t *iv, uint16_t iv_length, const uint8_t *associated_data, uint16_t associated_data_length, uint8_t *plain_data, uint32_t *plain_data_length)
Decrypt the encrypted data using symmetric encryption mode and export plain message to host.
@ OPTIGA_TLS12_PRF_SHA_512
Key derivation using TLSv1.2 PRF SHA512.
optiga_calc_ssec_params_t optiga_calc_ssec_params
calc ssec params
_STATIC_INLINE LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hkdf_sha256(optiga_crypt_t *me, uint16_t secret, const uint8_t *salt, uint16_t salt_length, const uint8_t *info, uint16_t info_length, uint16_t derived_key_length, bool_t export_to_host, uint8_t *derived_key)
Derives a key or shared secret using HKDF-SHA256 operation from the secret stored in OPTIGA.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_destroy(optiga_crypt_t *me)
Destroys an instance of optiga_crypt_t.
optiga_cmd_t * my_cmd
Command module instance.
enum optiga_hmac_type optiga_hmac_type_t
Specifies the HMAC generation types in OPTIGA.
enum optiga_symmetric_key_type optiga_symmetric_key_type_t
Specifies the symmetric key types supported by OPTIGA.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_symmetric_decrypt_final(optiga_crypt_t *me, const uint8_t *encrypted_data, uint32_t encrypted_data_length, uint8_t *plain_data, uint32_t *plain_data_length)
Decrypts input data using symmetric key from OPTIGA, exports block aligned plain data and completes t...
Specifies the data structure for ECDSA signature verification.
enum optiga_key_id optiga_key_id_t
Specifies the key location in OPTIGA.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_symmetric_decrypt_ecb(optiga_crypt_t *me, optiga_key_id_t symmetric_key_oid, const uint8_t *encrypted_data, uint32_t encrypted_data_length, uint8_t *plain_data, uint32_t *plain_data_length)
Decrypt the data using symmetric encryption scheme using ECB mode of operation.
_STATIC_INLINE LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hkdf_sha384(optiga_crypt_t *me, uint16_t secret, const uint8_t *salt, uint16_t salt_length, const uint8_t *info, uint16_t info_length, uint16_t derived_key_length, bool_t export_to_host, uint8_t *derived_key)
Derives a key or shared secret using HKDF-SHA384 operation from the secret stored in OPTIGA.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_symmetric_encrypt_start(optiga_crypt_t *me, optiga_symmetric_encryption_mode_t encryption_mode, optiga_key_id_t symmetric_key_oid, const uint8_t *plain_data, uint32_t plain_data_length, const uint8_t *iv, uint16_t iv_length, const uint8_t *associated_data, uint16_t associated_data_length, uint16_t total_plain_data_length, uint8_t *encrypted_data, uint32_t *encrypted_data_length)
Initiates a symmetric encryption sequence for input data using symmetric key from OPTIGA.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_symmetric_encrypt_final(optiga_crypt_t *me, const uint8_t *plain_data, uint32_t plain_data_length, uint8_t *encrypted_data, uint32_t *encrypted_data_length)
Encrypts input data using symmetric key from OPTIGA, exports block aligned encrypted data and complet...
Specifies the structure for asymmetric encryption and decryption.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hash_finalize(optiga_crypt_t *me, optiga_hash_context_t *hash_ctx, uint8_t *hash_output)
Finalizes and exports the hash output.
uint8_t bool_t
Typedef for a boolean.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hash(optiga_crypt_t *me, optiga_hash_type_t hash_algorithm, uint8_t source_of_data_to_hash, const void *data_to_hash, uint8_t *hash_output)
Updates a hashing for input data and returns digest.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_ecdsa_verify(optiga_crypt_t *me, const uint8_t *digest, uint8_t digest_length, const uint8_t *signature, uint16_t signature_length, uint8_t public_key_source_type, const void *public_key)
Verifies the signature over the given digest.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_ecc_generate_keypair(optiga_crypt_t *me, optiga_ecc_curve_t curve_id, uint8_t key_usage, bool_t export_private_key, void *private_key, uint8_t *public_key, uint16_t *public_key_length)
Generates a key pair based on ECC curves.
callback_handler_t handler
Callback handler.
_STATIC_INLINE LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_tls_prf_sha512(optiga_crypt_t *me, uint16_t secret, const uint8_t *label, uint16_t label_length, const uint8_t *seed, uint16_t seed_length, uint16_t derived_key_length, bool_t export_to_host, uint8_t *derived_key)
Derives a key using TLS PRF SHA512.
Specifies the data structure for ECDSA signature.
uint16_t instance_state
To provide the busy/free status of the crypt instance.
This file defines APIs, types and data structures used in the Command (cmd) module implementation.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_symmetric_encrypt_continue(optiga_crypt_t *me, const uint8_t *plain_data, uint32_t plain_data_length, uint8_t *encrypted_data, uint32_t *encrypted_data_length)
Encrypts input data using symmetric key from OPTIGA and exports block aligned encrypted data.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hash_start(optiga_crypt_t *me, optiga_hash_context_t *hash_ctx)
Initializes a hash context.
@ OPTIGA_TLS12_PRF_SHA_384
Key derivation using TLSv1.2 PRF SHA384.
optiga_derive_key_params_t optiga_derive_key_params
derive key params
Specifies the data structure for generate key pair.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_rsa_verify(optiga_crypt_t *me, optiga_rsa_signature_scheme_t signature_scheme, const uint8_t *digest, uint8_t digest_length, const uint8_t *signature, uint16_t signature_length, uint8_t public_key_source_type, const void *public_key, uint16_t salt_length)
Verifies the RSA signature over the given digest.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_rsa_sign(optiga_crypt_t *me, optiga_rsa_signature_scheme_t signature_scheme, const uint8_t *digest, uint8_t digest_length, optiga_key_id_t private_key, uint8_t *signature, uint16_t *signature_length, uint16_t salt_length)
Generates a RSA signature for the given digest based on the input signature scheme.
void(* callback_handler_t)(void *callback_ctx, optiga_lib_status_t event)
typedef for event callback handler
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hash_update(optiga_crypt_t *me, optiga_hash_context_t *hash_ctx, uint8_t source_of_data_to_hash, const void *data_to_hash)
Updates a hash context with the input data.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hmac_verify(optiga_crypt_t *me, optiga_hmac_type_t type, uint16_t secret, const uint8_t *input_data, uint32_t input_data_length, const uint8_t *hmac, uint32_t hmac_length)
Performs the HMAC verification for the provided authorization value using OPTIGA.
_STATIC_INLINE LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_tls_prf_sha384(optiga_crypt_t *me, uint16_t secret, const uint8_t *label, uint16_t label_length, const uint8_t *seed, uint16_t seed_length, uint16_t derived_key_length, bool_t export_to_host, uint8_t *derived_key)
Derives a key using TLS PRF SHA384.
union for OPTIGA crypt parameters
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_ecdsa_sign(optiga_crypt_t *me, const uint8_t *digest, uint8_t digest_length, optiga_key_id_t private_key, uint8_t *signature, uint16_t *signature_length)
Generates a signature for the given digest.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_symmetric_encrypt(optiga_crypt_t *me, optiga_symmetric_encryption_mode_t encryption_mode, optiga_key_id_t symmetric_key_oid, const uint8_t *plain_data, uint32_t plain_data_length, const uint8_t *iv, uint16_t iv_length, const uint8_t *associated_data, uint16_t associated_data_length, uint8_t *encrypted_data, uint32_t *encrypted_data_length)
Encrypt the data using symmetric encryption mode and export encrypted message to host.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_clear_auto_state(optiga_crypt_t *me, uint16_t secret)
This operation clears the AUTO state at OPTIGA for input secret OID.
optiga_gen_symkey_params_t optiga_gen_sym_key_params
generate symmetric key params
optiga_calc_hash_params_t optiga_calc_hash_params
calc hash params
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_rsa_encrypt_session(optiga_crypt_t *me, optiga_rsa_encryption_scheme_t encryption_scheme, const uint8_t *label, uint16_t label_length, uint8_t public_key_source_type, const void *public_key, uint8_t *encrypted_message, uint16_t *encrypted_message_length)
Encrypts session data using RSA public key.
_STATIC_INLINE LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_hkdf_sha512(optiga_crypt_t *me, uint16_t secret, const uint8_t *salt, uint16_t salt_length, const uint8_t *info, uint16_t info_length, uint16_t derived_key_length, bool_t export_to_host, uint8_t *derived_key)
Derives a key or shared secret using HKDF-SHA512 operation from the secret stored in OPTIGA.
uint16_t optiga_lib_status_t
typedef for OPTIGA host library status
@ OPTIGA_HKDF_SHA_512
Key derivation using HKDF-SHA512.
LIBRARY_EXPORTS optiga_lib_status_t optiga_crypt_symmetric_decrypt_continue(optiga_crypt_t *me, const uint8_t *encrypted_data, uint32_t encrypted_data_length, uint8_t *plain_data, uint32_t *plain_data_length)
Decrypts input encrypted data using symmetric key from OPTIGA and exports block aligned plain data.
Specifies the structure for derivation of key using pseudo random function.
optiga_get_random_params_t optiga_get_random_params
get random params