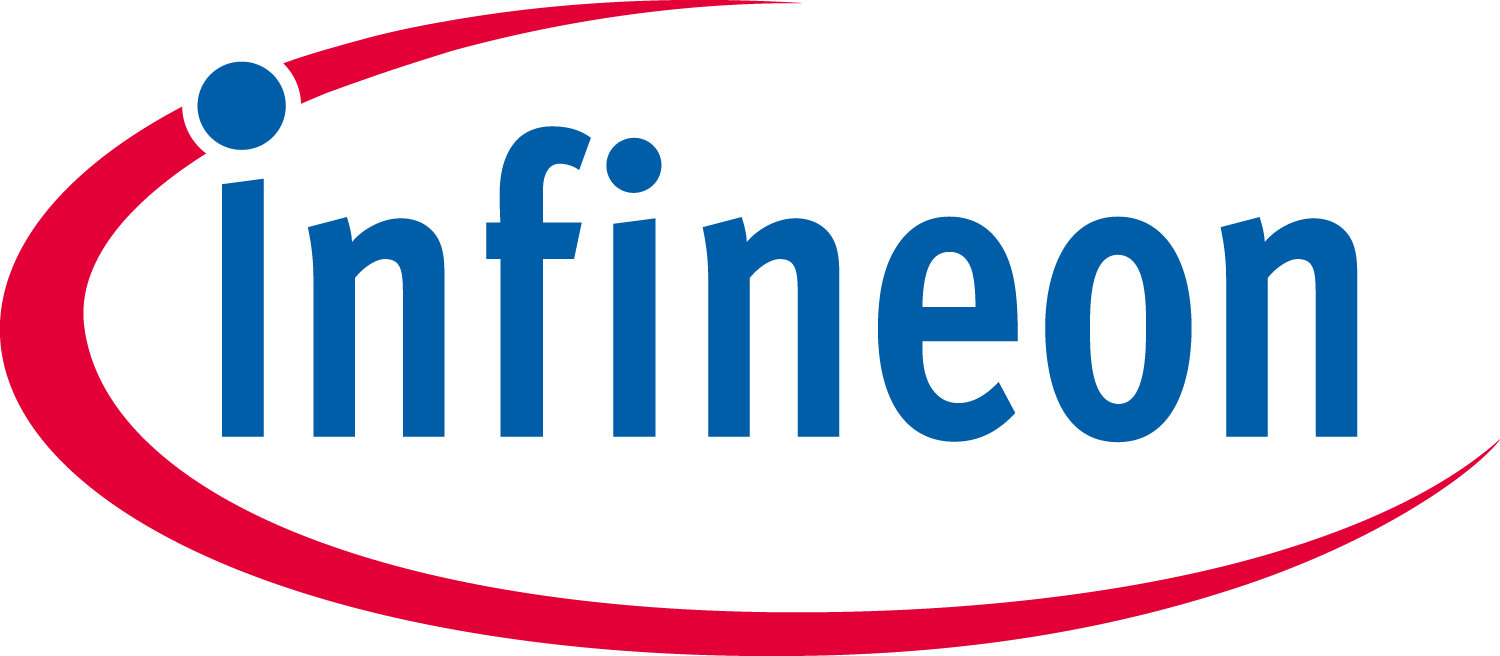 |
OPTIGA™ Trust M
Host Library Documentation
|
Go to the documentation of this file.
38 #ifndef _OPTIGA_LIB_COMMON_H_
39 #define _OPTIGA_LIB_COMMON_H_
50 #define OPTIGA_INSTANCE_ID_0 (0x00)
53 #define OPTIGA_COMMS_NO_PROTECTION (0x00)
55 #define OPTIGA_COMMS_COMMAND_PROTECTION (0x01)
57 #define OPTIGA_COMMS_RESPONSE_PROTECTION (0x02)
59 #define OPTIGA_COMMS_FULL_PROTECTION (0x03)
61 #define OPTIGA_COMMS_RE_ESTABLISH (0x80)
63 #define OPTIGA_COMMS_PROTOCOL_VERSION_PRE_SHARED_SECRET (0x01)
66 #define OPTIGA_CRYPT_HOST_DATA (0x01)
68 #define OPTIGA_CRYPT_OID_DATA (0x00)
71 #define OPTIGA_LIB_INSTANCE_BUSY (0x0001)
73 #define OPTIGA_LIB_INSTANCE_FREE (0x0000)
75 #ifdef OPTIGA_COMMS_SHIELDED_CONNECTION
77 #define OPTIGA_COMMS_PROTECTION_LEVEL (0x01)
79 #define OPTIGA_COMMS_PROTOCOL_VERSION (0x02)
101 #ifdef OPTIGA_CRYPT_SYM_GENERATE_KEY_ENABLED
144 #ifdef OPTIGA_CRYPT_ECC_NIST_P_521_ENABLED
148 #ifdef OPTIGA_CRYPT_ECC_BRAINPOOL_P_R1_ENABLED
188 #ifdef OPTIGA_CRYPT_RSA_SSA_SHA512_ENABLED
194 #if defined (OPTIGA_CRYPT_SYM_ENCRYPT_ENABLED) || defined (OPTIGA_CRYPT_SYM_DECRYPT_ENABLED) || \
195 defined (OPTIGA_CRYPT_HMAC_ENABLED) || defined (OPTIGA_CRYPT_HMAC_VERIFY_ENABLED)
241 #ifdef OPTIGA_CRYPT_HMAC_ENABLED
256 #ifdef OPTIGA_CRYPT_HKDF_ENABLED
278 #ifdef OPTIGA_CRYPT_TLS_PRF_SHA384_ENABLED
282 #ifdef OPTIGA_CRYPT_TLS_PRF_SHA512_ENABLED
288 #ifdef OPTIGA_CRYPT_SYM_GENERATE_KEY_ENABLED
541 #if defined (OPTIGA_CRYPT_RSA_ENCRYPT_ENABLED) || defined (OPTIGA_CRYPT_RSA_DECRYPT_ENABLED)
579 #if defined (OPTIGA_CRYPT_SYM_ENCRYPT_ENABLED) || defined (OPTIGA_CRYPT_SYM_DECRYPT_ENABLED)
623 #ifdef OPTIGA_CRYPT_SYM_GENERATE_KEY_ENABLED
675 uint16_t two_byte_value);
695 uint32_t four_byte_value);
715 uint16_t* p_two_byte_value);
Specifies the data structure for ecdh secret generation.
Specifies the data structure for symmetric encrypt and decrypt.
bool_t export_hash_ctx
export hash ctx
This file is defines the compilation switches to build code with required features.
uint16_t label_length
Label length.
enum optiga_key_usage optiga_key_usage_t
Specifies the key usage type in OPTIGA.
optiga_hash_context_t * p_hash_context
Context buffer pointer.
uint16_t bytes_to_read
Data size to be read.
@ OPTIGA_RNG_TYPE_DRNG
Generate Random data using DRNG.
const uint8_t * random_data
Random Seed/Salt.
const uint8_t * p_protected_update_buffer
Pointer to the buffer which contains manifest/fragment.
uint16_t derived_key_length
Derived Key length.
bool_t store_in_session
Use to indicate to acquire session.
@ OPTIGA_ECC_CURVE_NIST_P_384
Generate elliptic curve key based on NIST P384.
uint32_t received_data_length
Length of received data.
uint8_t * random_data
User buffer for storing random data.
optiga_symmetric_encryption_mode
Specifies the symmetric encryption schemes type in OPTIGA.
This file defines the error codes for the all the layers and modules. OPTIGA host library return val...
enum optiga_rsa_key_type optiga_rsa_key_type_t
Specifies the RSA key type in OPTIGA.
struct optiga_calc_sign optiga_calc_sign_params_t
Specifies the data structure for ECDSA signature.
@ OPTIGA_ECC_CURVE_BRAIN_POOL_P_384R1
Generate elliptic curve key based on ECC Brainpool 384R1.
uint16_t written_size
Contains length of data written in across multiple transceive calls. Used for chaining purpose.
enum optiga_rsa_signature_scheme optiga_rsa_signature_scheme_t
Specifies the RSA signature schemes type in OPTIGA.
struct optiga_set_data_object optiga_set_data_object_params_t
Specifies the data structure for data to be written to OPTIGA.
uint8_t digest_length
Digest data length.
uint16_t accumulated_size
Contains length of data received in across multiple transceive calls. Used for chaining purpose.
@ OPTIGA_HKDF_SHA_384
Key derivation using HKDF-SHA384.
@ OPTIGA_HMAC_SHA_512
Generated MAC using HMAC-SHA512.
optiga_set_obj_protected_tag
Specifies the set protected object tag.
enum optiga_hkdf_type optiga_hkdf_type_t
Specifies the HKDF key derivation types in OPTIGA.
optiga_key_id_t private_key
OID of the Private Key (either Key store or Session based). Refer optiga_key_id_t for possible values...
Specifies the data structure for data to be written to OPTIGA.
hash_data_in_optiga_t * p_hash_oid
OID hash pointer.
uint16_t random_data_length
Random data length.
Specifies the data structure for data to be read from OPTIGA.
uint8_t * p_signature
Signature buffer pointer.
enum optiga_symmetric_encryption_mode optiga_symmetric_encryption_mode_t
Specifies the symmetric encryption schemes type in OPTIGA.
@ OPTIGA_SYMMETRIC_CBC_MAC
Symmetric encryption mode with CBC_MAC mode.
@ OPTIGA_SET_PROTECTED_UPDATE_CONTINUE
This enables to continue of the protected update.
uint8_t * context_buffer
buffer to hold the hash context data
Specifies the structure to provide the details of data to be hashed from OPTIGA.
optiga_rsa_key_type
Specifies the RSA key type in OPTIGA.
optiga_hmac_type
Specifies the HMAC generation types in OPTIGA.
@ OPTIGA_HMAC_SHA_384
Generated MAC using HMAC-SHA384.
@ OPTIGA_HASH_TYPE_SHA_256
Hash algorithm type SHA256.
struct optiga_calc_ssec optiga_calc_ssec_params_t
Specifies the data structure for ecdh secret generation.
const void * key
Public key provided by host.
uint16_t message_length
Length of message to be encrypted. Set 0 if data from session OID.
uint8_t * derived_key
Pointer to a buffer where the exported key to be stored.
@ OPTIGA_RSASSA_PKCS1_V15_SHA256
Signature schemes RSA SSA PKCS1-v1.5 with SHA256 digest.
uint16_t oid
Object ID to be written.
optiga_hash_context_length
Specifies the hash context length in bytes.
Specifies the structure to the Hash context details managed by OPTIGA.
const uint8_t * associated_data
Pointer to associated data.
@ OPTIGA_ECC_CURVE_NIST_P_256
Generate elliptic curve key based on NIST P256.
public_key_from_host_t * public_key
Public Key of the peer.
const uint8_t * generated_hmac
Pointer to generated hmac.
Specifies the data structure of random generation.
@ OPTIGA_KEY_ID_E0FC
Key from key store for RSA (non-volatile)
optiga_key_id_t private_key_oid
OID of the Private Key (either Key store or Session based). Refer optiga_key_id_t for possible values...
uint16_t context_buffer_length
context length
optiga_key_usage
Specifies the key usage type in OPTIGA.
uint8_t count
Count value.
@ OPTIGA_SYMMETRIC_AES_256
Symmetric key type of AES-256.
struct optiga_get_random optiga_get_random_params_t
Specifies the data structure of random generation.
struct optiga_get_data_object optiga_get_data_object_params_t
Specifies the data structure for data to be read from OPTIGA.
This file contains the type definitions for the fundamental data types.
const uint8_t * message
Message to be encrypted. Set NULL if data from session OID.
optiga_symmetric_key_type
Specifies the symmetric key types supported by OPTIGA.
enum optiga_ecc_curve optiga_ecc_curve_t
Specifies the key curve type in OPTIGA.
uint8_t key_type
Public key type details. For ECC key use optiga_ecc_curve_t and for RSA key use optiga_rsa_key_type_t...
optiga_rsa_encryption_scheme
Specifies the RSA encryption schemes.
uint16_t oid
Object ID to be read.
enum optiga_hash_type optiga_hash_type_t
Specifies the hashing algorithm type in OPTIGA.
uint16_t size
Write data size.
@ OPTIGA_SYMMETRIC_CBC
Symmetric encryption mode with CBC mode.
uint16_t length
Length of public key buffer.
uint32_t data_sent
Data length has been sent.
uint32_t apparent_context_size
Possible context size to send in a fragment.
struct optiga_enc_dec_asym optiga_decrypt_asym_params_t
const uint8_t * p_digest
Digest buffer pointer.
uint8_t current_sequence
Variable to store current encrypt decrypt sequence.
Specifies the data structure for symmetric generate key.
Specifies the data structure of the Public Key details (key, size and type)
uint16_t optional_data_length
Optional data length.
uint8_t operation_mode
Symmetric mode of operation.
uint16_t total_input_data_length
Variable to indicate complete input data length required for CCM.
uint16_t input_shared_secret_oid
Session based (optiga_key_id_t) or Data object which has the pre-shared secret.
uint8_t mode
Encryption or hmac mode.
@ OPTIGA_KEY_ID_E0F1
Key from key store (non-volatile)
@ OPTIGA_TLS12_PRF_SHA_256
Key derivation using TLSv1.2 PRF SHA256.
uint8_t * p_out_digest
Out digest.
enum optiga_tls_prf_type optiga_tls_prf_type_t
Specifies the key derivation types.
void * symmetric_key
Symmetric key buffer pointer or oid pointer.
uint8_t * private_key
Private key buffer pointer.
bool_t export_symmetric_key
Symmetric key export option.
@ OPTIGA_SYMMETRIC_AES_192
Symmetric key type of AES-192.
uint16_t associated_data_length
Length of associated data.
bool_t export_private_key
Private key export option.
uint32_t generated_hmac_length
Length of generated hmac.
uint16_t offset
Offset within the data object.
struct hash_data_in_optiga hash_data_in_optiga_t
Specifies the structure to provide the details of data to be hashed from OPTIGA.
hash_data_from_host_t * p_hash_data
Data buffer pointer.
uint16_t offset
Offset of data with the object ID.
struct optiga_verify_sign optiga_verify_sign_params_t
Specifies the data structure for ECDSA signature verification.
struct public_key_from_host public_key_from_host_t
Specifies the data structure of the Public Key details (key, size and type)
uint8_t * buffer
Read data buffer pointer.
optiga_hash_type
Specifies the hashing algorithm type in OPTIGA.
uint8_t export_to_host
Export to Host (store in OPTIGA Session or export to host)
uint8_t public_key_source_type
Source of provided public key.
optiga_key_id_t private_key_oid
Type of public key OID.
enum optiga_rsa_encryption_scheme optiga_rsa_encryption_scheme_t
Specifies the RSA encryption schemes.
uint8_t data_or_metadata
Read to data or metadata.
uint32_t optiga_common_get_uint32(const uint8_t *p_input_buffer)
Prepares uint32 [Big endian] type value from the buffer and store.
enum optiga_rng_type optiga_rng_type_t
Specifies the random generation types.
Specifies the data structure of calculate hash.
@ OPTIGA_RNG_TYPE_TRNG
Generate Random data using TRNG.
@ OPTIGA_ECC_CURVE_NIST_P_521
Generate elliptic curve key based on ECC NIST P521.
uint16_t * processed_message_length
Pointer to the length of the encrypted or decrypted message.
@ OPTIGA_HKDF_SHA_256
Key derivation using HKDF-SHA256.
uint8_t data_or_metadata
Write to data or metadata.
@ OPTIGA_TLS12_PRF_SHA_512
Key derivation using TLSv1.2 PRF SHA512.
uint8_t manifest_version
manifest version
uint8_t key_usage
Key usage type.
enum optiga_hash_context_length optiga_hash_context_length_t
Specifies the hash context length in bytes.
@ OPTIGA_KEY_USAGE_AUTHENTICATION
This enables the private key for the signature generation as part of authentication commands.
const uint8_t * buffer
data to hash
@ OPTIGA_KEY_ID_SESSION_BASED
Key from session (volatile)
optiga_key_id
Specifies the key location in OPTIGA.
uint16_t offset
Offset of data with the object ID.
@ OPTIGA_ECC_CURVE_BRAIN_POOL_P_512R1
Generate elliptic curve key based on ECC Brainpool 512R1.
uint16_t last_read_size
Contains the data length received in last transceive. Used for chaining purpose.
uint16_t * private_key_length
Private key length.
struct optiga_symmetric_enc_dec_params optiga_encrypt_sym_params_t
Specifies the data structure for symmetric encrypt and decrypt.
enum optiga_hmac_type optiga_hmac_type_t
Specifies the HMAC generation types in OPTIGA.
enum optiga_symmetric_key_type optiga_symmetric_key_type_t
Specifies the symmetric key types supported by OPTIGA.
@ OPTIGA_HMAC_SHA_256
Generated MAC using HMAC-SHA256.
optiga_ecc_curve
Specifies the key curve type in OPTIGA.
const uint8_t * label
Label input as a constant string.
struct optiga_gen_keypair optiga_gen_keypair_params_t
Specifies the data structure for generate key pair.
Specifies the data structure for ECDSA signature verification.
enum optiga_key_id optiga_key_id_t
Specifies the key location in OPTIGA.
@ OPTIGA_KEY_USAGE_KEY_AGREEMENT
This enables the private key for key agreement (e.g. ecdh operations)
uint16_t random_data_length
Random Seed/Salt length.
uint8_t hash_sequence
Type of hash operation.
public_key_from_host_t * public_key
Public key provided by host.
Specifies the structure to provide the details of data to be hashed from host.
struct optiga_set_object_protected_params optiga_set_object_protected_params_t
Specifies the data structure for protected update.
uint32_t * out_data_length
Length of output data.
uint16_t signature_length
Signature data length.
@ OPTIGA_RSASSA_PKCS1_V15_SHA512
Signature schemes RSA SSA PKCS1-v1.5 with SHA512 digest.
@ OPTIGA_KEY_USAGE_ENCRYPTION
This enables the private key for encrypt and decrypt.
Specifies the structure for asymmetric encryption and decryption.
const uint8_t * info
Application specific info.
uint8_t bool_t
Typedef for a boolean.
@ OPTIGA_KEY_ID_E0F3
Key from key store (non-volatile)
uint16_t * ref_bytes_to_read
Pointer to the read buffer length.
struct optiga_symmetric_enc_dec_params optiga_decrypt_sym_params_t
struct hash_data_from_host hash_data_from_host_t
Specifies the structure to provide the details of data to be hashed from host.
optiga_set_obj_protected_tag_t set_obj_protected_tag
Set protected object tag.
uint16_t oid
OID of data object.
uint8_t digest_length
Digest data length.
Specifies the data structure for protected update.
uint16_t symmetric_key_oid
Symmetric key OID.
Specifies the data structure for ECDSA signature.
optiga_tls_prf_type
Specifies the key derivation types.
@ OPTIGA_SYMMETRIC_AES_128
Symmetric key type of AES-128.
uint8_t write_type
Type of write - Write only or Erase and write.
@ OPTIGA_SYMMETRIC_CMAC
Symmetric encryption mode with CMAC mode.
uint8_t key_usage
Key usage type.
@ OPTIGA_RSA_KEY_1024_BIT_EXPONENTIAL
Generate 1024 bit RSA key.
optiga_hkdf_type
Specifies the HKDF key derivation types in OPTIGA.
@ OPTIGA_TLS12_PRF_SHA_384
Key derivation using TLSv1.2 PRF SHA384.
uint32_t sent_data_length
Length of sent data.
uint16_t * p_signature_length
Signature length.
optiga_key_id_t private_key_id
Store private key OID.
@ OPTIGA_HASH_CONTEXT_LENGTH_SHA_256
Hash context length (in bytes) in case of SHA256.
uint16_t iv_length
Length of initialization vector.
uint8_t * out_data
Pointer to output data.
Specifies the data structure for generate key pair.
void optiga_common_set_uint16(uint8_t *p_output_buffer, uint16_t two_byte_value)
Copies 2 bytes of uint16 type value to the buffer.
uint8_t * processed_message
Pointer to buffer where encrypted or decrypted message is stored.
uint32_t length
Length of data.
uint16_t p_protected_update_buffer_length
Manifest/Fragment length.
uint32_t in_data_length
Length of plain text.
const uint8_t * p_signature
Signature buffer pointer.
@ OPTIGA_KEY_ID_E0FD
Key from key store for RSA (non-volatile)
@ OPTIGA_KEY_ID_E0F0
Key from key store (non-volatile)
@ OPTIGA_RSASSA_PKCS1_V15_SHA384
Signature schemes RSA SSA PKCS1-v1.5 with SHA384 digest.
uint8_t * public_key
Pointer to Public Key.
struct optiga_calc_hash optiga_calc_hash_params_t
Specifies the data structure of calculate hash.
@ OPTIGA_SET_PROTECTED_UPDATE_FINAL
This enables to finish of the protected update.
uint8_t current_hash_sequence
Current type of hash operation.
const uint8_t * in_data
Pointer to plain text.
optiga_rng_type
Specifies the random generation types.
uint8_t hash_algo
hashing algorithm
uint16_t length
Number of data bytes starting from the offset.
@ OPTIGA_SYMMETRIC_ECB
Symmetric encryption mode with ECB mode.
@ OPTIGA_RSA_KEY_2048_BIT_EXPONENTIAL
Generate 2048 bit RSA key.
uint8_t * shared_secret
Pointer to a buffer where the exported shared secret to be stored.
struct optiga_derive_key optiga_derive_key_params_t
Specifies the structure for derivation of key using pseudo random function.
@ OPTIGA_SET_PROTECTED_UPDATE_START
This enables to start of the protected update.
@ OPTIGA_KEY_ID_E0F2
Key from key store (non-volatile)
@ OPTIGA_ECC_CURVE_BRAIN_POOL_P_256R1
Generate elliptic curve key based on ECC Brainpool 256R1.
const uint8_t * buffer
Wrtie data buffer pointer.
struct optiga_enc_dec_asym optiga_encrypt_asym_params_t
Specifies the structure for asymmetric encryption and decryption.
uint8_t original_sequence
Requested sequence.
void optiga_common_get_uint16(const uint8_t *p_input_buffer, uint16_t *p_two_byte_value)
Prepares uint16 [Big endian] type value from the buffer and stores in the output pointer.
@ OPTIGA_KEY_USAGE_SIGN
This enables the private key for the signature generation.
uint8_t * public_key
Public key buffer pointer.
enum optiga_set_obj_protected_tag optiga_set_obj_protected_tag_t
Specifies the set protected object tag.
uint16_t * public_key_length
Public key length.
optiga_rsa_signature_scheme
Specifies the RSA signature schemes type in OPTIGA.
@ OPTIGA_RSAES_PKCS1_V15
RSA PKCS1 v1.5 encryption scheme.
uint16_t certificate_oid
Public key certificate OID.
@ OPTIGA_HKDF_SHA_512
Key derivation using HKDF-SHA512.
struct optiga_gen_symkey_params optiga_gen_symkey_params_t
Specifies the data structure for symmetric generate key.
uint8_t public_key_source_type
Source of provided public key for encryption and Private key for decryption.
@ OPTIGA_KEY_ID_SECRET_BASED
Key from key store for symmetric operations.
const uint8_t * p_digest
Digest buffer pointer.
const uint8_t * iv
Pointer to initialization vector.
const uint8_t * optional_data
User buffer which holds the optional data.
void optiga_common_set_uint32(uint8_t *p_output_buffer, uint32_t four_byte_value)
Copies 4 bytes of uint32 [Big endian] type value to the buffer and stores in the output pointer.
struct optiga_hash_context optiga_hash_context_t
Specifies the structure to the Hash context details managed by OPTIGA.
Specifies the structure for derivation of key using pseudo random function.
uint16_t info_length
Info length.