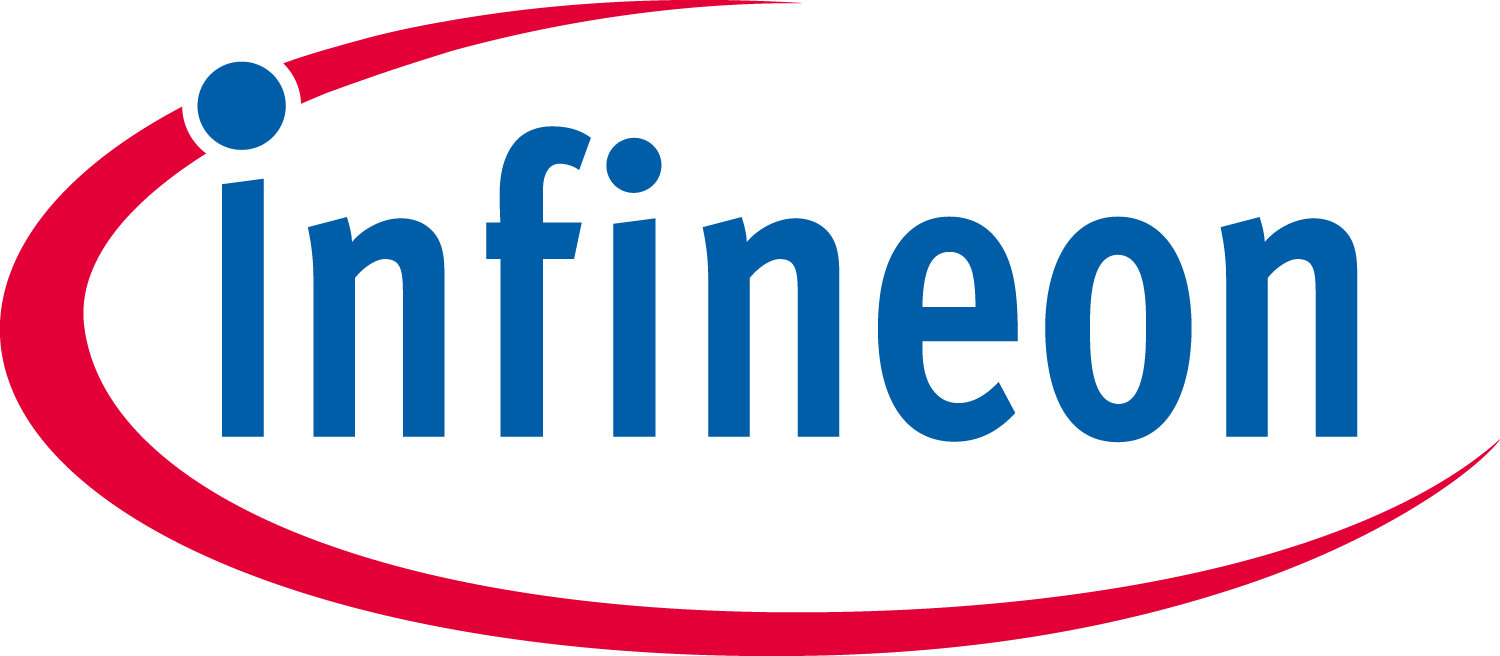 |
OPTIGA™ Trust M
Host Library Documentation
|
Go to the documentation of this file.
38 #ifndef _OPTIGA_LIB_TYPES_H_
39 #define _OPTIGA_LIB_TYPES_H_
51 #ifdef _OPTIGA_EXPORTS_DLLEXPORT_H_
53 #ifdef OPTIGA_LIB_EXPORTS
54 #define LIBRARY_EXPORTS __declspec(dllexport)
56 #define LIBRARY_EXPORTS __declspec(dllimport)
57 #endif // OPTIGA_LIB_EXPORTS
60 #define LIBRARY_EXPORTS
114 #define _STATIC_H static
119 #ifndef _STATIC_INLINE
120 #define _STATIC_INLINE static inline
void(* upper_layer_callback_t)(void *upper_layer_ctx, optiga_lib_status_t event)
typedef for application event handler
float float_t
Typedef for a float.
Structure to specify a byte stream consisting of length and data pointer.
uint8_t * data_ptr
Pointer to byte array which contains the data stream.
struct data_blob data_blob_t
Structure to specify a byte stream consisting of length and data pointer.
char char_t
Typedef for one byte integer.
void * hdl_t
typedef for handles
uint16_t length
Length of the byte stream.
uint8_t bool_t
Typedef for a boolean.
double double_t
Typedef for a double word.
void(* callback_handler_t)(void *callback_ctx, optiga_lib_status_t event)
typedef for event callback handler
void Void
Typedef for a void.
uint16_t optiga_lib_status_t
typedef for OPTIGA host library status