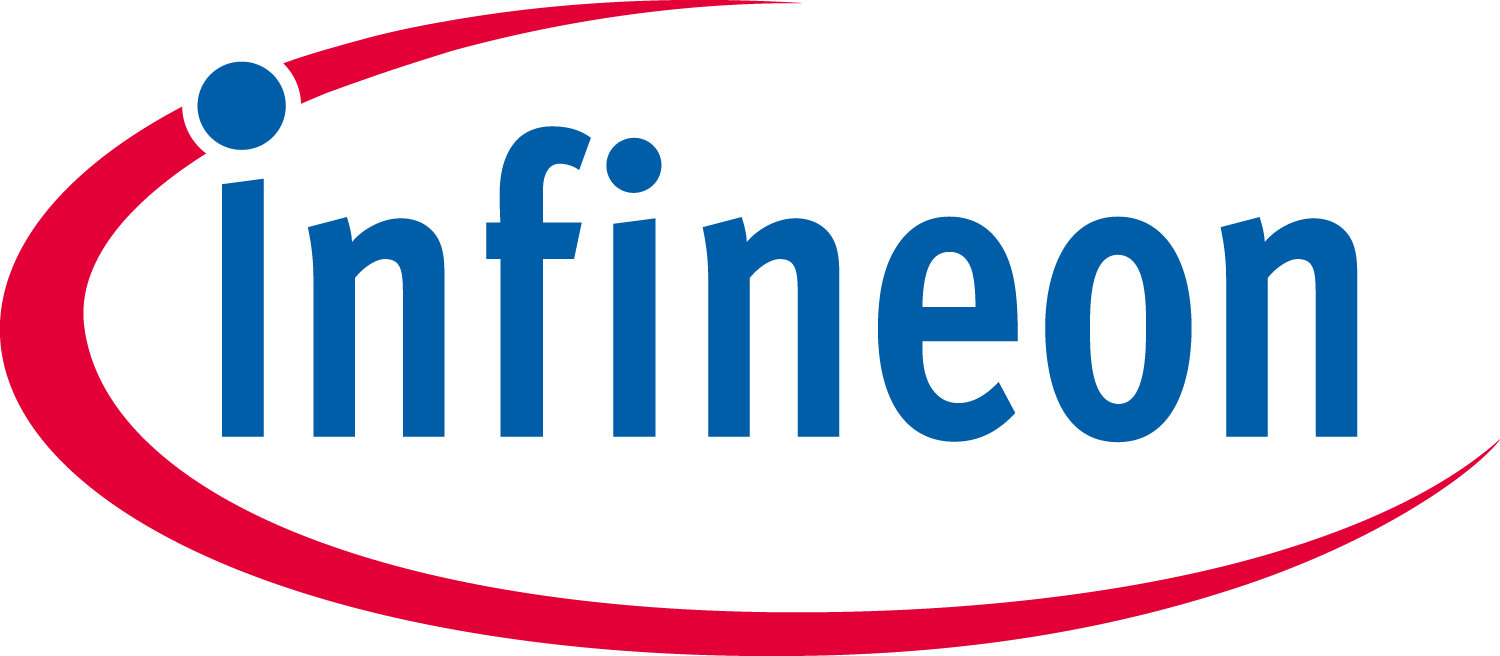 |
OPTIGA™ Trust M
Host Library Documentation
|
Go to the documentation of this file.
38 #ifndef _IFX_I2C_CONFIG_H_
39 #define _IFX_I2C_CONFIG_H_
45 #ifdef IFX_I2C_CONFIG_EXTERNAL
46 #include "ifx_i2c_config_external.h"
57 #define IFX_I2C_BASE_ADDR (0x30)
60 #define PL_POLLING_INVERVAL_US (1000U)
62 #define PL_POLLING_MAX_CNT (200U)
64 #define PL_DATA_POLLING_INVERVAL_US (5000U)
66 #define PL_GUARD_TIME_INTERVAL_US (50U)
71 #ifdef IFX_I2C_FRAME_SIZE
72 #if (IFX_I2C_FRAME_SIZE > 277) || (IFX_I2C_FRAME_SIZE < 16)
73 #error "Unsupported value for IFX_I2C_FRAME_SIZE"
77 #define IFX_I2C_FRAME_SIZE (277U)
81 #define TL_HEADER_SIZE (1U)
83 #define DL_HEADER_SIZE (5U)
85 #define DL_TRANS_REPEAT (3U)
87 #define PL_TRANS_TIMEOUT_MS (10U)
90 #define TL_MAX_EXIT_TIMEOUT (180U)
93 #define RESET_LOW_TIME_MSEC (2000U)
95 #define STARTUP_TIME_MSEC (12000U)
98 #define IFX_I2C_STACK_SUCCESS (0x0000)
100 #define IFX_I2C_STACK_BUSY (0x0001)
102 #define IFX_I2C_STACK_ERROR (0x0102)
104 #define IFX_I2C_STACK_MEM_ERROR (0x0104)
106 #define IFX_I2C_FATAL_ERROR (0x0106)
108 #define IFX_I2C_HANDSHAKE_ERROR (0x0107)
110 #define IFX_I2C_SESSION_ERROR (0x0108)
113 #define IFX_I2C_DL_HEADER_OFFSET (0U)
115 #define IFX_I2C_TL_HEADER_OFFSET (IFX_I2C_DL_HEADER_OFFSET + 3)
117 #define IFX_I2C_LOG_PL (0U)
119 #define IFX_I2C_LOG_DL (0U)
121 #define IFX_I2C_LOG_TL (0U)
123 #define IFX_I2C_LOG_PRL (0U)
126 #define IFX_I2C_LOG_ID_PL (0x00)
128 #define IFX_I2C_LOG_ID_DL (0x01)
130 #define IFX_I2C_LOG_ID_TL (0x02)
132 #define IFX_I2C_LOG_ID_PRL (0x03)
134 #define IFX_I2C_LOG_ID_PAL (0x04)
136 #if defined OPTIGA_COMMS_SHIELDED_CONNECTION
137 #define IFX_I2C_TL_ENABLE (1U)
138 #define IFX_I2C_PRL_ENABLED (1U)
140 #define IFX_I2C_PRESENCE_BIT (0x08)
141 #define IFX_I2C_PRL_MAC_SIZE (0x08)
142 #define IFX_I2C_PRL_HEADER_SIZE (0x05)
143 #define IFX_I2C_PRL_OVERHEAD_SIZE (IFX_I2C_PRL_HEADER_SIZE + IFX_I2C_PRL_MAC_SIZE)
145 #define IFX_I2C_DATA_OFFSET (IFX_I2C_PRL_HEADER_SIZE)
147 #define IFX_I2C_PRESENCE_BIT_CHECK (0x08)
149 #define IFX_I2C_PRESENCE_BIT (0x00)
150 #define IFX_I2C_PRL_MAC_SIZE (0x00)
151 #define IFX_I2C_PRL_HEADER_SIZE (0x00)
152 #define IFX_I2C_PRL_OVERHEAD_SIZE (IFX_I2C_PRL_HEADER_SIZE + IFX_I2C_PRL_MAC_SIZE)
154 #define IFX_I2C_DATA_OFFSET (IFX_I2C_PRL_HEADER_SIZE)
156 #define IFX_I2C_PRESENCE_BIT_CHECK (0x00)
160 #define PROTOCOL_VERSION_PRE_SHARED_SECRET (0x01)
162 #define IFX_I2C_SESSION_CONTEXT_RESTORE (0x11)
164 #define IFX_I2C_SESSION_CONTEXT_SAVE (0x22)
166 #define IFX_I2C_SESSION_CONTEXT_NONE (0x33)
169 #define NO_PROTECTION (0x00)
171 #define MASTER_PROTECTION (0x01)
173 #define SLAVE_PROTECTION (0x02)
175 #define FULL_PROTECTION (0x03)
177 #define RE_ESTABLISH (0x80)
180 #define IFX_I2C_SESSION_KEY_BUFFER_SIZE (0x28)
188 const uint8_t * data,
309 #if defined OPTIGA_COMMS_SHIELDED_CONNECTION
312 typedef struct ifx_i2c_prl_manage_context
317 uint8_t decryption_failure_counter;
319 uint8_t data_retransmit_counter;
321 uint8_t negotiation_state;
323 uint8_t stored_context_flag;
325 uint32_t master_sequence_number;
327 uint32_t save_slave_sequence_number;
328 }ifx_i2c_prl_manage_context_t;
331 typedef struct ifx_i2c_datastore_config
334 uint8_t protocol_version;
336 uint16_t datastore_shared_secret_id;
338 uint16_t datastore_manage_context_id;
340 uint16_t shared_secret_length;
341 } ifx_i2c_datastore_config_t;
345 typedef struct ifx_i2c_prl
352 uint8_t negotiation_state;
356 uint32_t master_sequence_number;
358 uint32_t slave_sequence_number;
360 uint32_t save_slave_sequence_number;
362 uint8_t * p_actual_payload;
364 uint16_t actual_payload_length;
366 uint8_t * p_recv_payload_buffer;
368 uint16_t * p_recv_payload_buffer_length;
376 uint8_t prl_header_offset;
382 uint8_t prl_txrx_buffer[58];
384 uint16_t prl_txrx_receive_length;
386 uint8_t associate_data[8];
388 uint16_t prl_receive_length;
390 uint8_t decryption_failure_counter;
392 uint8_t data_retransmit_counter;
396 uint8_t restore_context_flag;
398 ifx_i2c_prl_manage_context_t prl_saved_ctx;
402 uint8_t trans_repeat_status;
421 #if defined OPTIGA_COMMS_SHIELDED_CONNECTION
422 ifx_i2c_datastore_config_t * ifx_i2c_datastore_config;
447 #ifdef OPTIGA_COMMS_SHIELDED_CONNECTION
452 uint8_t protection_level;
454 uint8_t protocol_version;
456 uint8_t manage_context_operation;
464 #ifdef OPTIGA_COMMS_SHIELDED_CONNECTION
ifx_i2c_event_handler_t upper_layer_event_handler
This file is defines the compilation switches to build code with required features.
uint16_t * p_upper_layer_rx_buffer_len
Pointer to length of upper layer rx buffer.
#define IFX_I2C_SESSION_KEY_BUFFER_SIZE
Session key buffer size.
uint16_t actual_packet_length
Actual length of user provided packet.
void(* upper_layer_callback_t)(void *upper_layer_ctx, optiga_lib_status_t event)
typedef for application event handler
uint8_t register_action
Action on register, read/write.
This file provides the prototype declarations of PAL I2C.
upper_layer_callback_t upper_layer_event_handler
Upper layer event handler.
uint16_t packet_offset
Offset till which data is sent from p_actual_packet.
uint16_t buffer_tx_len
Tx length.
#define IFX_I2C_FRAME_SIZE
Data link layer: frame size (max supported is 277 in OPTIGA ).
uint8_t payload_offset
Tl rx payload copy offset.
uint8_t tx_seq_nr
Tx sequence number.
uint16_t retry_counter
Retry counter.
uint32_t frame_start_time
Start time of sending frame.
struct ifx_i2c_tl ifx_i2c_tl_t
Transport layer structure.
Physical layer structure.
uint16_t frame_size
Data link layer frame size.
uint32_t data_poll_timeout
Timeout value.
uint8_t retransmit_counter
Retransmit counter.
void * p_upper_layer_ctx
Upper layer context.
void(* ifx_i2c_event_handler_t)(struct ifx_i2c_context *p_ctx, optiga_lib_status_t event, const uint8_t *data, uint16_t data_len)
Event handler function prototype.
uint8_t * p_rx_frame_buffer
Pointer to main receive buffers.
pal_gpio_t * p_slave_reset_pin
Pointer to pal gpio context for reset.
uint16_t tx_buffer_size
Transmit buffer size.
optiga_lib_status_t close_state
Close states.
uint8_t * p_tx_frame_buffer
Pointer to main transmit buffers.
This file provides API prototypes of platform abstraction layer for datastore operations.
uint8_t frame_state
Frame state.
uint8_t * p_upper_layer_rx_buffer
Pointer to upper layer rx buffer.
pal_gpio_t * p_slave_vdd_pin
Pointer to pal gpio context for vdd.
uint8_t request_soft_reset
Soft reset requested.
PAL I2C context structure.
Transport layer structure.
Datalink layer structure.
ifx_i2c_context_t ifx_i2c_context_0
IFX I2C Instance.
uint8_t master_chaining_error_count
Chaining error count for master.
uint8_t tx_frame_buffer[IFX_I2C_FRAME_SIZE+1]
IFX I2C tx frame of max length.
uint8_t * p_actual_packet
Pointer to packet provided by user.
uint8_t frame_action
Action of frame. Tx/Rx.
This file provides the prototype declarations of PAL OS timer functionalities.
This file provides the prototype declarations of PAL GPIO.
uint8_t state
Datalink layer state.
ifx_i2c_event_handler_t upper_layer_event_handler
Upper layer event handler.
uint16_t * p_recv_packet_buffer_length
Length of receive buffer.
optiga_lib_status_t error_event
Error event state.
struct ifx_i2c_pl ifx_i2c_pl_t
Physical layer structure.
uint8_t reset_state
reset states
ifx_i2c_tl_t tl
Transport layer context.
This file provides the prototypes for the OPTIGA library logger.
uint8_t status
ifx i2c wrapper api status
uint16_t rx_buffer_size
Receive buffer size.
uint8_t reset_type
type of reset
uint8_t * p_recv_packet_buffer
Pointer to user provided receive buffer.
uint8_t action_rx_only
Indicate only Rx required.
struct ifx_i2c_dl ifx_i2c_dl_t
Datalink layer structure.
ifx_i2c_dl_t dl
Datalink layer context.
uint8_t do_pal_init
init pal
uint8_t negotiate_state
Negotiation state.
uint16_t total_recv_length
Total received data.
uint16_t max_packet_length
Maximum length of packet at transport layer.
pal_i2c_t * p_pal_i2c_ctx
Pointer to pal i2c context.
uint8_t i2c_cmd
i2c read/i2c write
uint8_t state
Transport layer state.
uint16_t frequency
Frequency of i2c master.
uint8_t rx_frame_buffer[IFX_I2C_FRAME_SIZE+1]
IFX I2C rx frame of max length.
IFX I2C context structure.
uint16_t tx_frame_len
Length of data to be sent.
uint8_t error
Error occured.
uint8_t tx_payload_offset
Tl tx payload copy offset.
uint16_t buffer_rx_len
Rx length.
Structure defines the PAL GPIO configuration.
ifx_i2c_event_handler_t upper_layer_event_handler
uint8_t * p_tx_frame
Pointer to data to be sent.
uint8_t buffer[IFX_I2C_FRAME_SIZE+1]
Physical layer buffer.
struct ifx_i2c_context ifx_i2c_context_t
IFX I2C context structure.
uint8_t chaining_error_count
Chaining error count from slave.
uint8_t slave_address
I2C Slave address.
uint8_t previous_chaining
State to check last chaining state.
uint32_t api_start_time
Start time of the transport layer API.
uint16_t optiga_lib_status_t
typedef for OPTIGA host library status
uint8_t transmission_completed
transmission done
uint8_t initialization_state
Initial state check.
ifx_i2c_pl_t pl
Physical layer context.
uint8_t resynced
Resynced.