Object and Metadata management
Objects
An object within this library is a generic class, which represents basic functionality and features valid for all OIDs (Object IDs, except for Session Objects) located on the chip.
Here are basic features what you can do with a generic Object instance (if access condition allows):
Read/write data from/to the object
Read/write metadata from/to the object
Below you can find object maps for various supported products
OPTIGA™ Trust M3
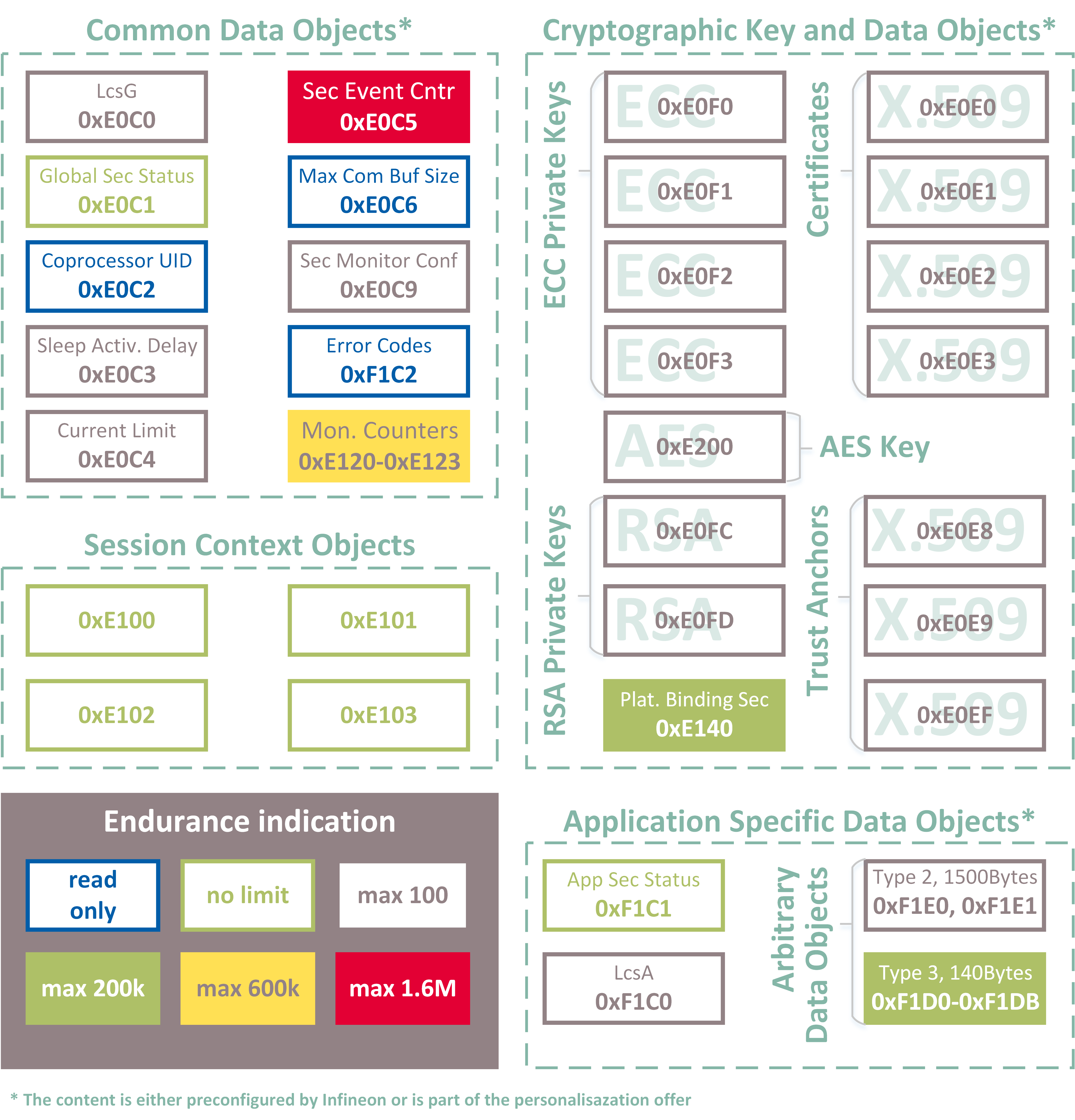
import optigatrust as optiga
# Create an instance of object.
object = optiga.Object(0xf1d0)
# Read data
object.read().hex()
Output
'01020304 ... 898a8b8c'
X509 object
from optigatrust import objects
cert_object = objects.X509(0xe0e0)
# Output the certificate content
print(cert_object)
Output
================== Certificate Object [0xe0e0] ==================
Lifecycle State :creation
Size :514
Access Condition: Read :always
Access Conditions: Change :never
PEM :
b'-----BEGIN CERTIFICATE-----
MIIB9TCCAXygAwIBAgIEcoMKdTAKBggqhkjOPQQDAzByMQswCQYDVQQGEwJERTEh
MB8GA1UECgwYSW5maW5lb24gVGVjaG5vbG9naWVzIEFHMRMwEQYDVQQLDApPUFRJ
R0EoVE0pMSswKQYDVQQDDCJJbmZpbmVvbiBPUFRJR0EoVE0pIFRydXN0IE0gQ0Eg
MzAwMB4XDTIwMDkxMDExNDAyNFoXDTQwMDkxMDExNDAyNFowGjEYMBYGA1UEAwwP
SW5maW5lb25Jb1ROb2RlMFkwEwYHKoZIzj0CAQYIKoZIzj0DAQcDQgAEIWaWiiWP
xWts8JdGXH819hVKg3IHVIvhrC5WWJhR2tyxVP3LuqRXjHJCVwDQHJfQ3z5hm1UA
PTDk5I/zlwbEWaNYMFYwDgYDVR0PAQH/BAQDAgCAMAwGA1UdEwEB/wQCMAAwFQYD
VR0gBA4wDDAKBggqghQARAEUATAfBgNVHSMEGDAWgBSzg+GsVpQGWa/Yr1cheEV0
jgxJmTAKBggqhkjOPQQDAwNnADBkAjA9YBGLJmvpUdyQ8WiviQPg9pTyIFzBNpUb
ZdLarO1Gu44zvMhFoeR/dtnjahgv59UCMHBNfeU+ZFfbk7B+fRHrrotyBSt63GcE
efqXeARctwDp9bStWx8d9JOw9B0h4mnfTA==
-----END CERTIFICATE-----'
Issuer: Common Name :Infineon OPTIGA(TM) Trust M CA 300
Subject: Common Name :InfineonIoTNode
Public Key :042166968a258fc56b6cf097465c7f35f6154a837 ... 01c97d0df3e619b55003d30e4e48ff39706c459
Signature :306402303d60118b266be951dc90f168af8903e0f ... cb700e9f5b4ad5b1f1df493b0f41d21e269df4c
============================================================
ECC Key object
from optigatrust import objects
import json
key_object = objects.ECCKey(0xe0f1)
print(json.dumps(key_object.meta, indent=4))
Output
{
"lcso": "creation",
"change": [
"lcso",
"<",
"operational"
],
"execute": "always",
"algorithm": "secp256r1",
"key_usage": [
"authentication",
"signature"
]
}
RSA Key object
from optigatrust import objects
import json
key_object = objects.RSAKey(0xe0fc)
print(json.dumps(key_object.meta, indent=4))
Output
{
"lcso": "creation",
"change": [
"lcso",
"<",
"operational"
],
"execute": "always",
"algorithm": "rsa2048",
"key_usage": [
"signature",
"key_agreement"
]
}
Metadata
Every Object (except for Session Objects) have a special service area called - metadata. It is a structured data which can significantly influence many aspects of the object itself, for example a lifecycle state.
Options
Users can work with metadata directly in human-readable form. Metadata should be contructed in the following manner:
{'<metadata_tag>': '<metadata_value>'}
Where metadata can take the following values:
Access Conditions
The ‘<metadata_value>’ should be defined either as a list of values; e.g.
- a constructed value
{‘read’: [‘lcso’, ‘<’, ‘operational’]}
Read is allowed only if the lifecycle state of the object is less than operational
- a more complex constructed value
{‘read’: [‘lcso’, ‘<’, ‘operational’, ‘&&’, ‘conf’, ‘0xe1’, ‘0x40’]}
Read is allowed only if the lifecycle state of the object is less than operation and under shielded connection (using e140 OID as a binding secret)
Tag |
Meaning |
---|---|
‘always’ |
an action (for instance ‘read’) is always allowed |
‘never’ |
an action (for instance ‘read’) is forbidden |
‘lcsg’ |
Global Lifecycle State (Object ID 0xe0c0) this tag is used as a first argument for a complex expressions; e.g. [‘lcsg’, ‘<’, ‘operational’] |
‘lcsa’ |
Application Lifecycle State (Object ID 0xf1c0) this tag is used as a first argument for a complex expressions; e.g. [‘lcsa’, ‘<’, ‘operational’] |
‘lcso’ |
Object Lifecycle State (it is part of the metadata, see the section above) this tag is used as a first argument for a complex expressions; e.g. [‘lcso’, ‘<’, ‘operational’] |
‘conf’ |
|
‘int’ |
|
‘auto’ |
|
‘luc’ |
|
‘sec_sta_g’ |
x |
‘sec_sta_a’ |
x |
‘==’ |
“If equal”. Typical values [‘lcso’, ‘==’, ‘initialization’] |
‘>’ |
“If more”. Typical values [‘lcso’, ‘>’, ‘initialization’] |
‘<’ |
“If less”. Typical values [‘lcso’, ‘<’, ‘initialization’] |
‘&&’ |
“And”. Typical values [‘lcso’, ‘<’, ‘initialization’, ‘&&’, ‘conf’, ‘0xe1’, ‘0x40’] |
‘||’ |
“or”. Typical values [‘lcso’, ‘<’, ‘initialization’, ‘&&’, ‘conf’, ‘0xe1’, ‘0x40’] |
Printing All metadata in human readable form
from optigatrust import x509
import json
cert_object = x509.Certificate(0xe0e0)
print(json.dumps(cert_object.meta, indent=4))
Updating Metadata of the Certificate Object
from optigatrust import x509
cert_object = x509.Certificate(0xe0e0)
# Print out certificate sucessfully
print(cert_object)
# Store old metadata from the chip
old_meta = cert_object.meta['read']
# Prepare new metadata to write on the chip
new_meta = {'read': 'never'}
# Update metadata on the chip
cert_object.meta = new_meta
# Try to read-out the content one more time. Result: Value Error
print(cert_object)
# Revert the metadata
cert_object.meta = old_meta
# See that printing is again possible
print(cert_object)
Output
C:\Users\User\git\python-optiga-trust>python
Python 3.8.1 (tags/v3.8.1:1b293b6, Dec 18 2019, 22:39:24) [MSC v.1916 32 bit (Intel)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> from optigatrust import x509
>>> cert_object = x509.Certificate(0xe0e0)
Loaded: liboptigatrust-libusb-win-i686.dll
================== OPTIGA Trust Chip Info ==================
Firmware Identifier [dwFirmwareIdentifier]:0x80101071
Build Number [rgbESWBuild]:0x809
Current Limitation [OID: 0xE0C4]:0xf
Sleep Activation Delay [OID: 0xE0C3]:0x14
Global Lifecycle State [OID: 0xE0C0]:operational
Security Status [OID: 0xE0C1]:0x0
Security Event Counter [OID: 0xE0C5]:0x0
============================================================
>>> print(cert_object)
================== Certificate Object [0xe0e0] ==================
Lifecycle State :creation
Size :485
Access Condition: Read :always
Access Conditions: Change :never
PEM :
b'-----BEGIN CERTIFICATE-----
MIIB2DCCAX6gAwIBAgIEa9mwITAKBggqhkjOPQQDAjByMQswCQYDVQQGEwJERTEh
MB8GA1UECgwYSW5maW5lb24gVGVjaG5vbG9naWVzIEFHMRMwEQYDVQQLDApPUFRJ
R0EoVE0pMSswKQYDVQQDDCJJbmZpbmVvbiBPUFRJR0EoVE0pIFRydXN0IE0gQ0Eg
MTAxMB4XDTE5MDYxODA2MjgyM1oXDTM5MDYxODA2MjgyM1owHDEaMBgGA1UEAwwR
SW5maW5lb24gSW9UIE5vZGUwWTATBgcqhkjOPQIBBggqhkjOPQMBBwNCAATxjN/R
RdonS92dwYnvIIZnD0HrIhYg9lcZsSv3urXRnjL/xVEs/ijCzWKCQruY2CsTv2jg
iemizjIl4jURWfa9o1gwVjAOBgNVHQ8BAf8EBAMCAIAwDAYDVR0TAQH/BAIwADAV
BgNVHSAEDjAMMAoGCCqCFABEARQBMB8GA1UdIwQYMBaAFDwwjFzViuijXTKA5FSD
sv/Nhk0jMAoGCCqGSM49BAMCA0gAMEUCIDdnY4NzhosPawMGoY138J6uHzsLU6nZ
euBnZOnwnlwzAiEAkXneR8Gzkrk1S6f9zva8J6fe8LYejW15eXOwDyt7Nss=
-----END CERTIFICATE-----'
Issuer: Common Name :Infineon OPTIGA(TM) Trust M CA 101
Subject: Common Name :Infineon IoT Node
Public Key :04f18cdfd145da274bdd9dc189ef2086670f41eb221620f65719b12bf7bab5d19e32ffc5512cfe28c2cd628242bb98d82b13bf68e089e9a2ce3225e2351159f6bd
Signature :304502203767638373868b0f6b0306a18d77f09eae1f3b0b53a9d97ae06764e9f09e5c330221009179de47c1b392b9354ba7fdcef6bc27a7def0b61e8d6d797973b00f2b7b36cb
============================================================
>>> old_meta = cert_object.meta['read']
>>> new_meta = {'read': 'never'}
>>> cert_object.meta = new_meta
>>> print(cert_object)
Error: 0x8007
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "C:\Users\Yushev\git\python-optiga-trust\optigatrust\cert.py", line 613, in __str__
pem = '{0:<30}:\n{1}\n'.format("PEM", str(self.pem).replace('\\n', '\n').replace('\\t', '\t'))
File "C:\Users\Yushev\git\python-optiga-trust\optigatrust\cert.py", line 642, in pem
pem_cert += _break_apart(base64.b64encode(self.der).decode(), '\n', 64)
File "C:\Users\Yushev\git\python-optiga-trust\optigatrust\cert.py", line 632, in der
self._der = self._read()
File "C:\Users\Yushev\git\python-optiga-trust\optigatrust\cert.py", line 768, in _read
raise ValueError(
ValueError: Certificate Slot 57568 is empty
>>> cert_object.meta = old_meta
>>> print(cert_object)
================== Certificate Object [0xe0e0] ==================
Lifecycle State :creation
Size :485
Access Condition: Read :always
Access Conditions: Change :never
PEM :
b'-----BEGIN CERTIFICATE-----
MIIB2DCCAX6gAwIBAgIEa9mwITAKBggqhkjOPQQDAjByMQswCQYDVQQGEwJERTEh
MB8GA1UECgwYSW5maW5lb24gVGVjaG5vbG9naWVzIEFHMRMwEQYDVQQLDApPUFRJ
R0EoVE0pMSswKQYDVQQDDCJJbmZpbmVvbiBPUFRJR0EoVE0pIFRydXN0IE0gQ0Eg
MTAxMB4XDTE5MDYxODA2MjgyM1oXDTM5MDYxODA2MjgyM1owHDEaMBgGA1UEAwwR
SW5maW5lb24gSW9UIE5vZGUwWTATBgcqhkjOPQIBBggqhkjOPQMBBwNCAATxjN/R
RdonS92dwYnvIIZnD0HrIhYg9lcZsSv3urXRnjL/xVEs/ijCzWKCQruY2CsTv2jg
iemizjIl4jURWfa9o1gwVjAOBgNVHQ8BAf8EBAMCAIAwDAYDVR0TAQH/BAIwADAV
BgNVHSAEDjAMMAoGCCqCFABEARQBMB8GA1UdIwQYMBaAFDwwjFzViuijXTKA5FSD
sv/Nhk0jMAoGCCqGSM49BAMCA0gAMEUCIDdnY4NzhosPawMGoY138J6uHzsLU6nZ
euBnZOnwnlwzAiEAkXneR8Gzkrk1S6f9zva8J6fe8LYejW15eXOwDyt7Nss=
-----END CERTIFICATE-----'
Issuer: Common Name :Infineon OPTIGA(TM) Trust M CA 101
Subject: Common Name :Infineon IoT Node
Public Key :04f18cdfd145da274bdd9dc189ef2086670f41eb221620f65719b12bf7bab5d19e32ffc5512cfe28c2cd628242bb98d82b13bf68e089e9a2ce3225e2351159f6bd
Signature :304502203767638373868b0f6b0306a18d77f09eae1f3b0b53a9d97ae06764e9f09e5c330221009179de47c1b392b9354ba7fdcef6bc27a7def0b61e8d6d797973b00f2b7b36cb
============================================================
API
- class optigatrust.Object(object_id)
A class used to represent an Object on the OPTIGA Trust Chip
- Parameters:
id (int) – the id of the object; e.g. 0xe0e0
updated (bool) – This boolean variable notifies whether metadata or data has been updated and this can bu used to notify other modules to reread data if needed
- property meta
A dictionary of the metadata present right now on the chip for the given object. It is writable, so user can update the metadata assigning the value to it. Example return
{ "lcso": "creation", "change": [ "lcso", "<", "operational" ], "execute": "always", "algorithm": "secp384r1", "key_usage": "0x21" }
- read(offset=0, force=False) bytearray
This function helps to read the data stored on the chip
- Parameters:
offset (int) – An optional parameter defining whether you want to read the data with offset
force – This is a
bool
parameter which can be used to try to read the data even if id can’t be found
- Raises:
ValueError - when any of the parameters contain an invalid value
TypeError - when any of the parameters are of the wrong type
OSError - when an error is returned by the chip initialisation library
- Returns:
byte string
- write(data, offset=0)
This function helps to write the data onto the chip
- Parameters:
data – byte string to write
offset (int) – An optional parameter defining whether you want to read the data with offset
- :raises
ValueError - when any of the parameters contain an invalid value
TypeError - when any of the parameters are of the wrong type
OSError - when an error is returned by the chip initialisation library
This module implements all Object manipulation related APIs of the optigatrust package
- class optigatrust.objects.AESKey
A class used to represent an aes key object on the OPTIGA Trust Chip
- class optigatrust.objects.AcquiredSession
A class used to represent a session object on the OPTIGA Trust Chip. This is a pseudo object, just to indicate to OPTIGA, that we would like to use the acquired session
- class optigatrust.objects.AppData(object_id)
A class used to represent an Application Data object on the OPTIGA Trust Chip.
- class optigatrust.objects.ECCKey(key_id: int)
A class used to represent an ecc key object on the OPTIGA Trust Chip
- class optigatrust.objects.RSAKey(key_id: int)
A class used to represent an rsa key object on the OPTIGA Trust Chip
- class optigatrust.objects.Session(key_id: int)
A class used to represent a session object on the OPTIGA Trust Chip.
- class optigatrust.objects.X509(cert_id: int)
A class used to represent a certificate on the OPTIGA Trust Chip
- property der
This property allows to get or set the certificate in der form. Input should be a valid DER encoded certificate.
- property pem
This property allows to get or set the certificate in PEM form. Input should be a valid PEM formatted certificate.
- property pkey
This property allows to get the public key from the certificate. In case the certificate can’t be parsed an exception will be generated
- property signature
This property allows to get the signature of the certificate. In case the certificate can’t be parsed an exception will be generated