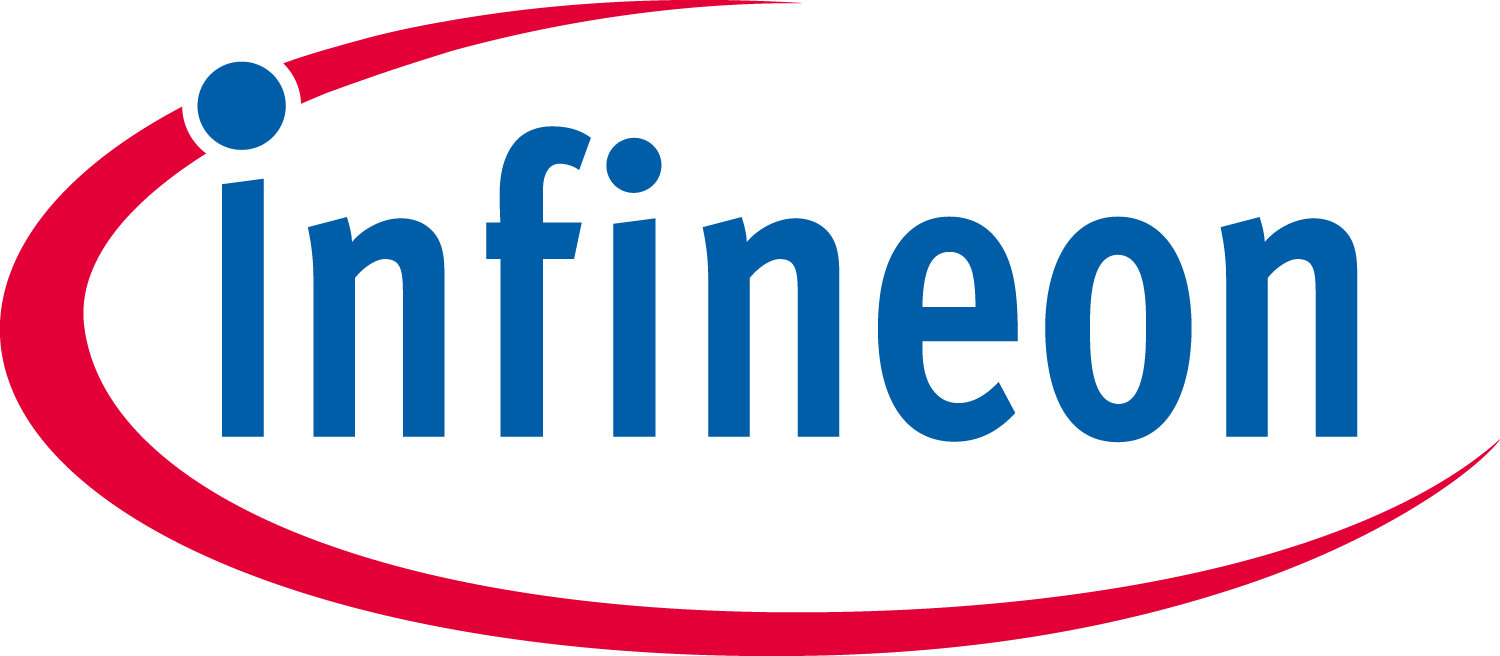 |
OPTIGA™ Trust M
Host Library Documentation
|
Go to the documentation of this file.
39 #ifndef _PROTECTED_UPDATE_PAL_CRYPTO_
40 #define _PROTECTED_UPDATE_PAL_CRYPTO_
58 uint8_t hash_algorithm,
59 const uint8_t * p_message,
60 uint32_t message_length,
66 uint16_t digest_length,
67 uint8_t * p_signature,
68 uint16_t * signature_length,
69 const uint8_t * p_private_key,
70 uint16_t private_key_length);
79 const uint8_t * p_plain_text,
80 uint16_t plain_text_length,
81 const uint8_t * p_encrypt_key,
82 const uint8_t * p_nonce,
83 uint16_t nonce_length,
84 const uint8_t * p_associated_data,
85 uint16_t associated_data_length,
87 uint8_t * p_cipher_text);
90 const uint8_t * p_secret,
91 uint16_t secret_length,
92 const uint8_t * p_label,
93 uint16_t label_length,
94 const uint8_t * p_seed,
96 uint8_t * p_derived_key,
97 uint16_t derived_key_length);
102 uint8_t * p_random_data ,
103 uint16_t random_data_length );
108 uint16_t seed_length);
113 uint8_t ** D, uint16_t * D_length,
114 uint8_t ** X, uint16_t * X_length,
115 uint8_t ** Y, uint16_t * Y_length);
118 uint8_t ** N, uint16_t * N_length,
119 uint8_t ** E, uint16_t * E_length,
120 uint8_t ** D, uint16_t * D_length);
122 #endif //_PROTECTED_UPDATE_PAL_CRYPTO_
This file defines APIs, types and data structures used for protected update data set creation.
PAL crypt context structure.
void * callback_ctx
callback
enum signature_algo signature_algo_t